On this post I am going to present you how to introduce reCaptcha in VueJS forms in a very easy way.
Google reCaptcha
What is Google reCAPTCHA? reCaptcha is a free service from Google that helps you to protect your websites from spam and abuse. A “CAPTCHA” is a turing test to tell human and bots apart. This test is easy for humans to solve, but hard for “bots” and other malicious software to figure out. By adding reCAPTCHA to a site, you can block automated software while helping your welcome users to enter with ease.
First of all, we need to create a Google reCaptcha instance under your Google account, by going to https://www.google.com/recaptcha/admin
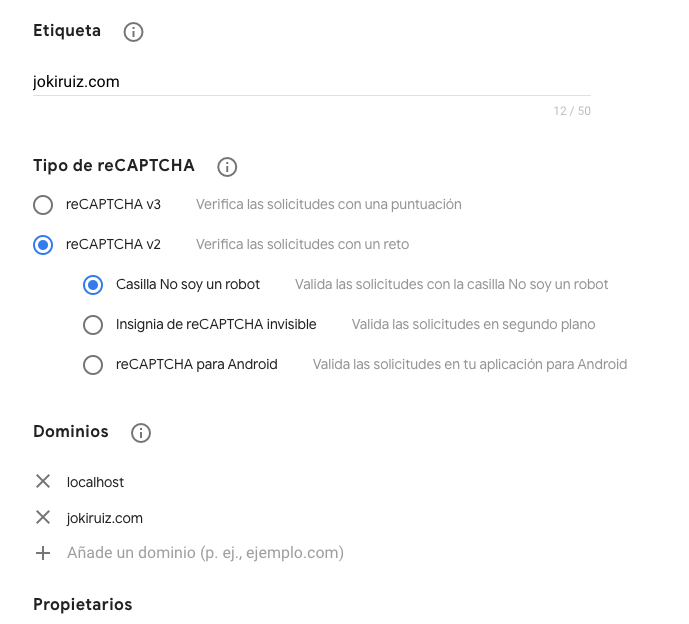
To learn more about reCAPTCHA, visit the official website and the technical documentation.
Creating our VueJS component
VueJS is a progressive framework for building user interfaces. The core library is focused on the view layer only, and is easy to pick up and integrate with other libraries or existing projects.
We have chosen this framework to create our form because I like its simplicity, performance and lightweight codebase, but we could have chosen other like ReactJS or Angular.
We will use a library called vue-recaptcha to ease the logic behind Google reCaptcha https://github.com/DanSnow/vue-recaptcha To install it using NPM, type:
npm install --save vue-recaptcha
Or via CDN:
<script src="https://unpkg.com/vue-recaptcha@latest/dist/vue-recaptcha.min.js"></script>
The template – HTML
Here is an example of a form component in VueJS. The form is quite standard, but you can see a <vue-recaptcha> tag, which is a vue component wrapper for reCaptcha.
<template>
<form :model="form" @submit.prevent="submit" ref="contactForm">
<div class="form-group row">
<label ...></label>
<div class="col-sm-10">
<input v-model="..." required="">
</div>
</div>
<div class="form-group row">
...
</div>
<div class="form-group row">
<label for="robot" class="col-sm-2 col-form-label"></label>
<div class="col-sm-10">
<vue-recaptcha ref="recaptcha"
@verify="onVerify" sitekey="/*/*/*/*/*/">
</vue-recaptcha>
</div>
</div>
<button type="submit" class="btn btn-primary mb-2">Send</button>.
</form>
</template>
The logic – JAVASCRIPT
Te core of our component lives in the next Javascript. If we take a look of the vue-recaptcha tag, we have passed in a handler call onVerify, that will be called once the user is verified, and the sitekey that we obtained when we registered the reCaptcha instance in Google.
import VueRecaptcha from 'vue-recaptcha';
export default {
data() {
return {
form: {
...
robot: false
}
}
},
methods: {
submit: function() {
if (this.form.robot) {
...
}
},
onVerify: function (response) {
if (response) this.form.robot = true;
},
},
components: {
'vue-recaptcha': VueRecaptcha
},
}
The logic is pretty simple, once the reCaptcha is verified, we change our form.robot prop, and when we click submit the form, we check whether our form.robot prop is true.
The result
You can see the result on my site https://jokiruiz.com/contact/
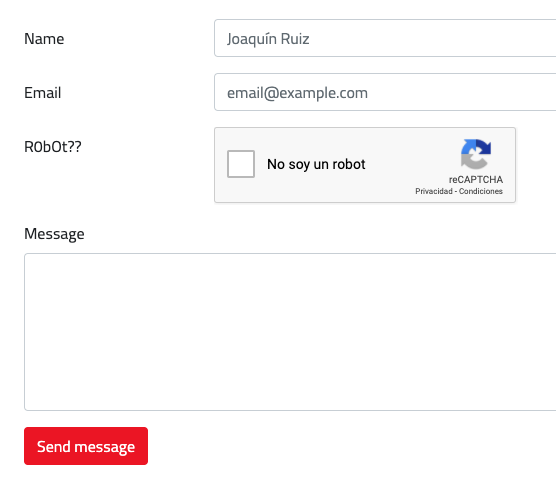
Did you develop the integration in a different way? Do you have problems installing reCaptcha in your form?
Share the post and Comment below! 🙂