It is very easy to display WordPress posts ordered by date, by name… But you might need to order or display them by views. How to do that?
It is easier than you think. You just need to do three things:
- to create a post_meta called for example “joki_post_views_count”
- increase this post_meta when someone visit your post
- add this post_meta on the WP_Query
Create a post_meta to track the visits of your WordPress posts
In order to do this, we need to add a function that we call each time someone visit our posts. You can do this on your theme’s functions.php file, or, as I recommend, inside a plugin.
function joki_count_views($postID) {
$post_meta = 'joki_post_views_count';
$count = get_post_meta($postID, $post_meta, true);
if($count == '') {
$count = 0;
delete_post_meta($postID, $post_meta);
add_post_meta($postID, $post_meta, '0');
}
else {
$count++;
update_post_meta($postID, $post_meta, $count);
}
}
In this function, we get the value of the post_meta ‘joki_post_views_count’ and if it doesn’t exist, we create it from 0, and if it exists, we increase its value.
Increase the post_meta when someone visit your post
For this, we need to identify one WordPress function that is executed every time someone visit one of our posts, that function is ‘wp_head’. Therefore we add an action to that function that calls the function we defined earlier. But this function is not only called when visits posts, so we need to check that.
function joki_track_views ($post_id) {
if ( !is_single() ) { return; }
if ( empty ( $postId) ) {
global $post;
$postId = $post->ID;
}
joki_count_views($postId);
}
add_action( 'wp_head', 'joki_track_views');
With the function “!is_single” we check whether we are in a post or somewhere else in WordPress.
Order WordPress posts by views
Once we have our posts with the post_meta “joki_post_views_count” updating, we just need to add the post_meta to the WordPress WP_Query query.
new WP_Query(
array(
'posts_per_page' => 6,
'meta_key' => 'joki_post_views_count',
'orderby' => 'meta_value_num',
'order' => 'DESC'
)
);
Learn how to use WP_Query on the official Codex site.
Extra / Remove the prefetching
There is a function executed on the wp_head called adjacent_posts_rel_link_wp_head , that displays relational links for the posts adjacent to the current post for single post pages.
In order to be more accurate with the counting, we should remove that function from wp_head.
remove_action( 'wp_head', 'adjacent_posts_rel_link_wp_head', 10);
Extra / Be careful with the Cache plugins
If you are caching the wp_head function with a Cache plugin, make sure you exclude or make dynamic the function ‘joki_count_views’.
Check if your counter is working
To check that your counter is working, you can use this query against your database:
SELECT * FROM `wp_postmeta` WHERE meta_key = 'joki_post_views_count';
And you should see something like:
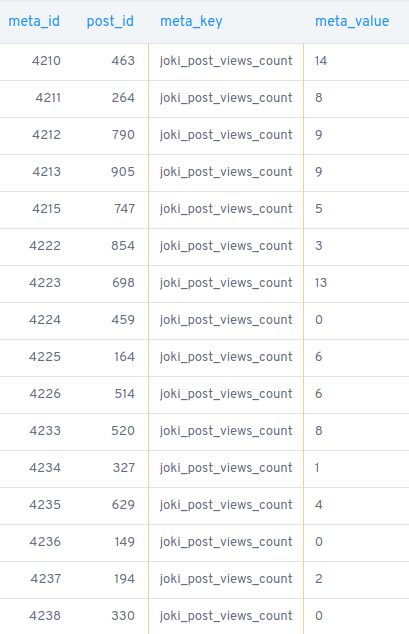